For stepper motor, I tried a lot of different things and failed. It took me a while to figure out what to do.
I first started with just setting up the motor. then I tried to set up motor with ESP32.
Then I tried to use sound sensor. I actually burnt one of the sound sensors.
I also tried to 3D print parts to make the motor move.
Moving motor
wiring
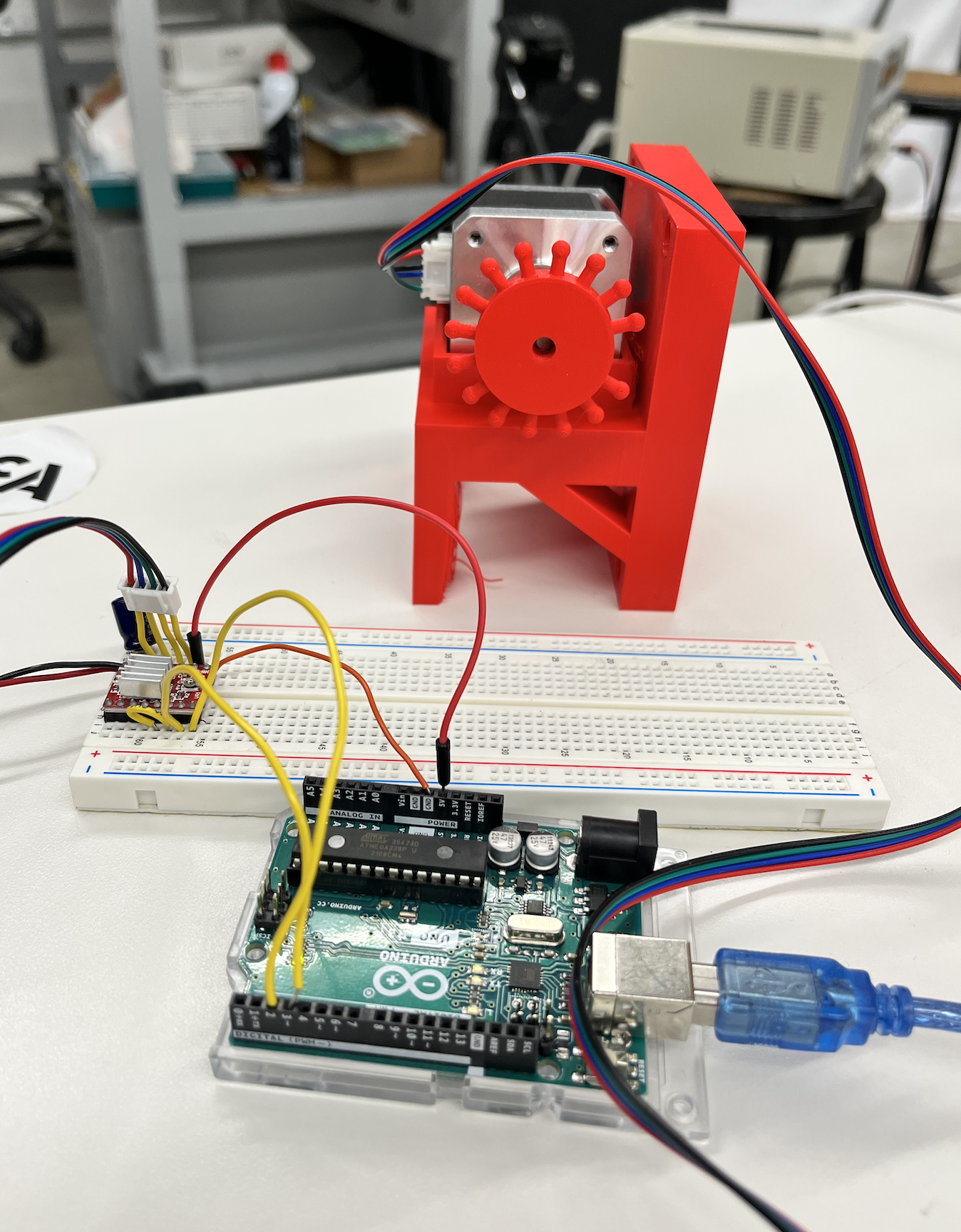
rotate board
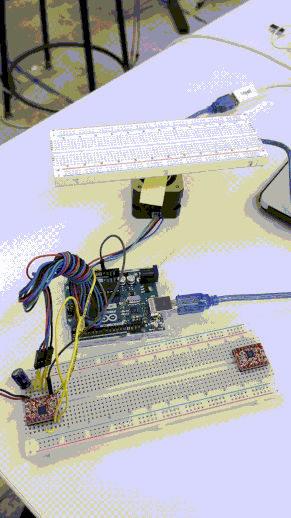
Arduino code for moving stepper motor
#include
const int stepPin = 13; // blue
const int dirPin = 12; // orange
// Define a stepper and the pins it will use
AccelStepper stepper(1, stepPin, dirPin); // initialise accelstepper for a two wire board
void setup()
{
}
void loop()
{
if (stepper.distanceToGo() == 0)
{
// Random change to speed, position and acceleration
// Make sure we dont get 0 speed or accelerations
delay(1000);
stepper.moveTo(rand() % 1000);
stepper.setMaxSpeed((rand() % 1000) + 1);
stepper.setAcceleration((rand() % 1000) + 1);
}
stepper.run();
}
ESP32 with Stepper Motor
Final Product
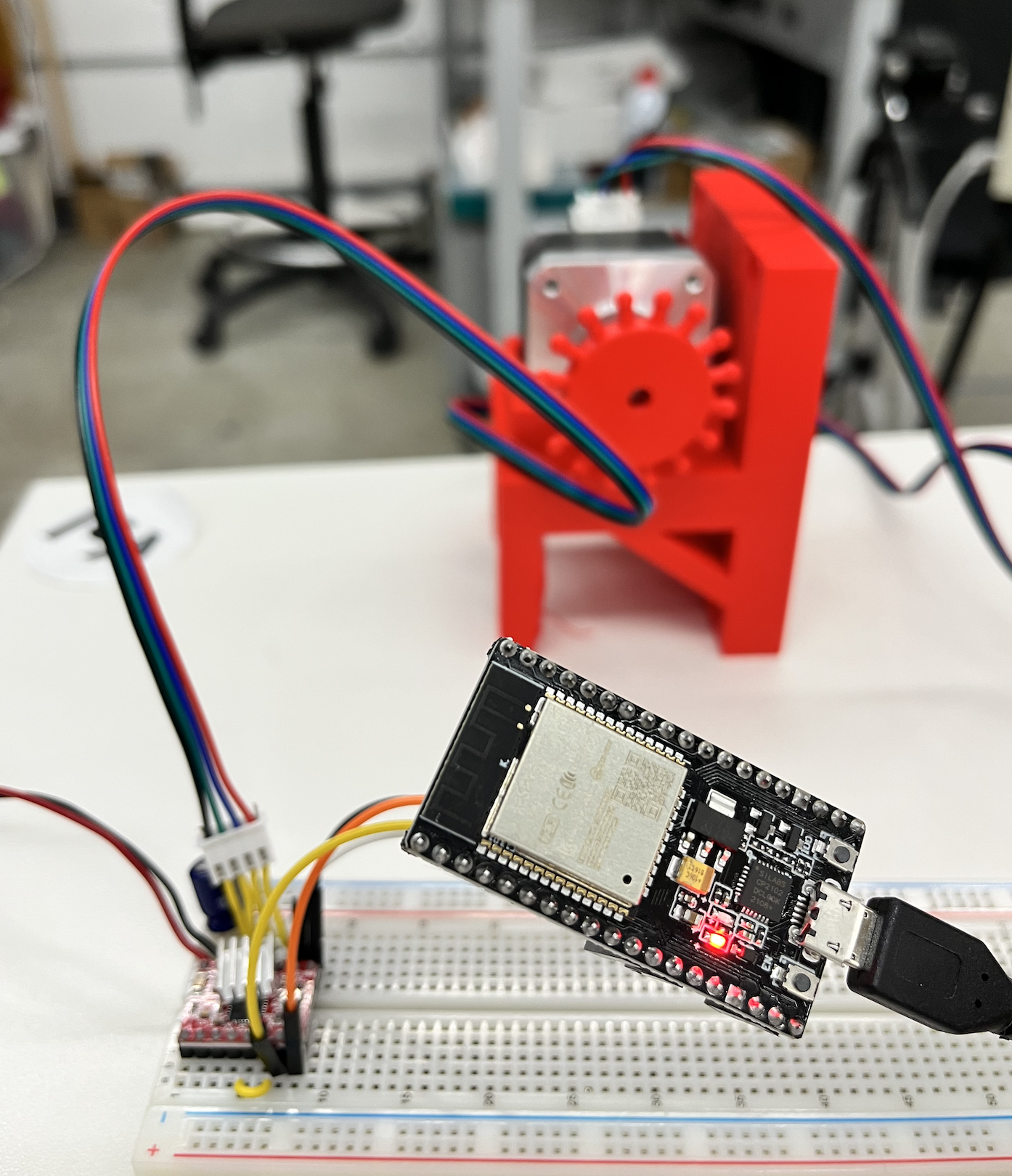
3D printed product
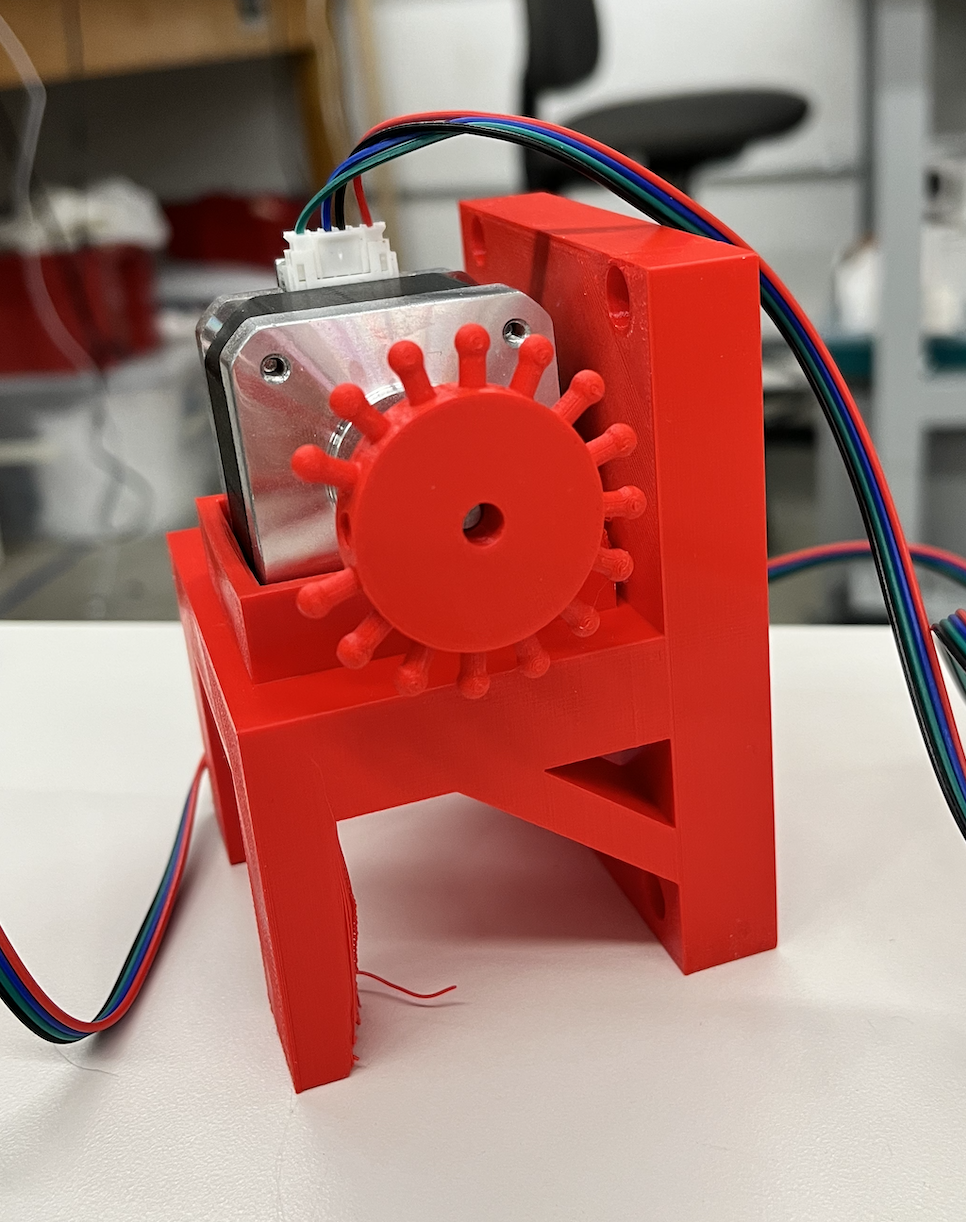
Arduino code for stepper motor ESP 32
#include
int in1Pin = 25;
int in2Pin = 33;
int in3Pin = 32;
int in4Pin = 35;
Stepper motor(512, in1Pin, in2Pin, in3Pin, in4Pin);
void setup()
{
pinMode(in1Pin, OUTPUT);
pinMode(in2Pin, OUTPUT);
pinMode(in3Pin, OUTPUT);
pinMode(in4Pin, OUTPUT);
// this line is for Leonardo's, it delays the serial interface
// until the terminal window is opened
//while (!Serial);
Serial.begin(115200);
motor.setSpeed(20);
}
void loop()
{
if (Serial.available())
{
int steps = Serial.parseInt();
motor.step(steps);
}
}
Sound Sensor
Final Product
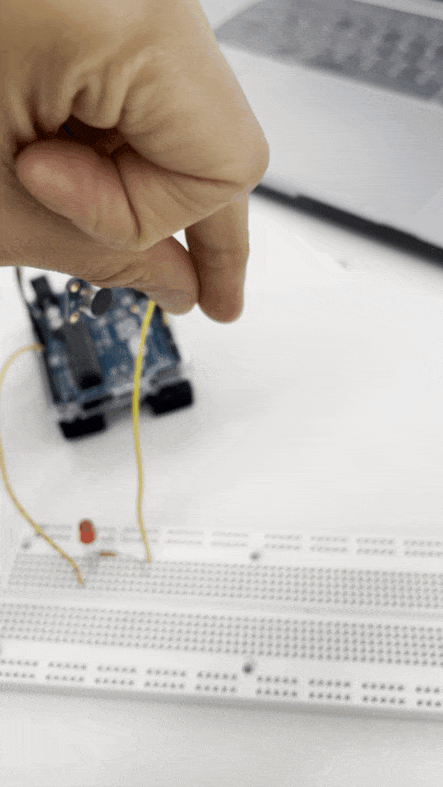
diagram wire
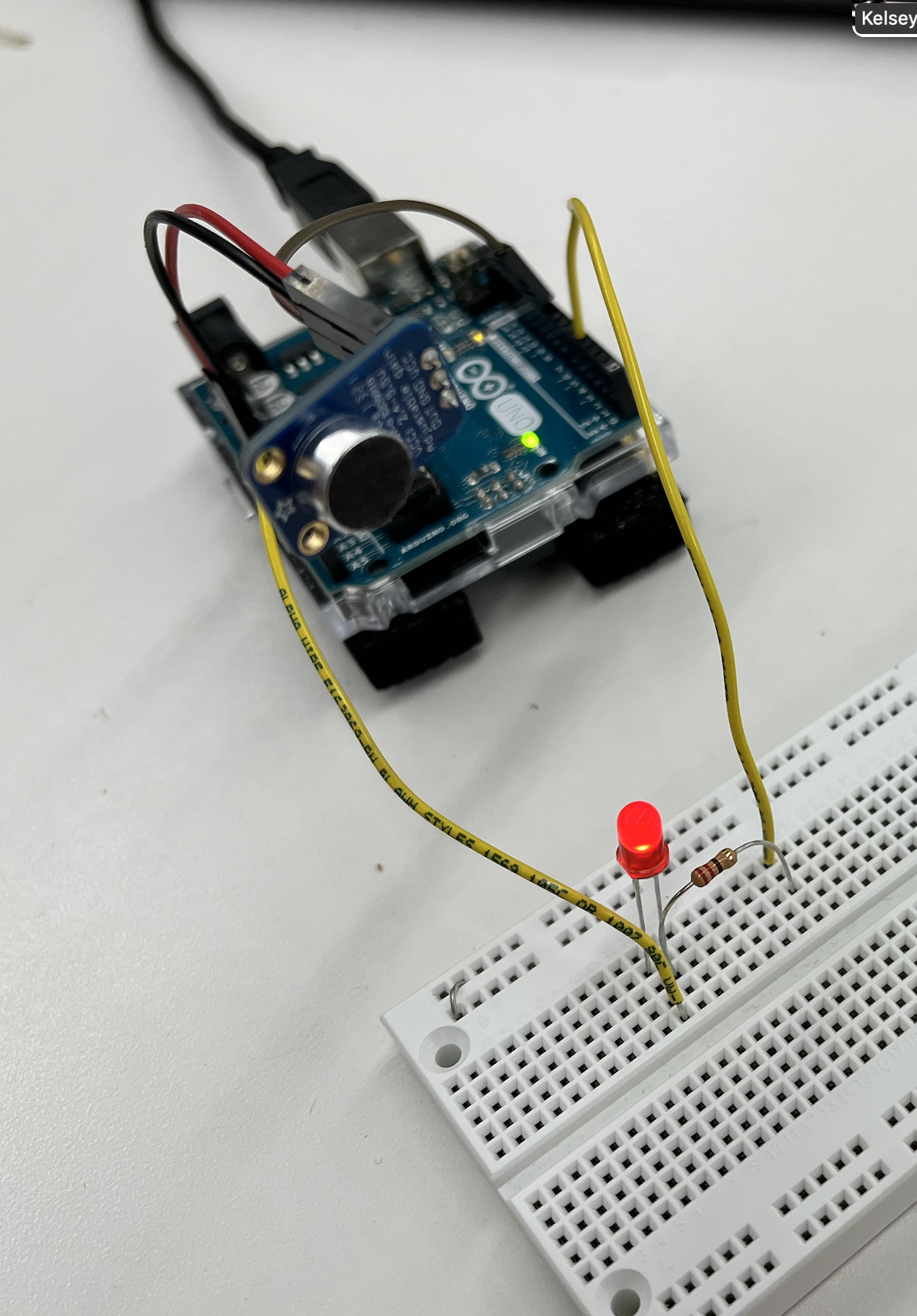
Arudino code for sound sensor
#define DO 8
#define LED_pin 4
unsigned long last_event = 0;
boolean LED_state = false;
void setup() {
pinMode(LED_pin, OUTPUT);
pinMode(DO, INPUT);
}
void loop() {
int output = digitalRead(DO);
if (output == LOW) {
if (millis() - last_event > 25) {
Serial.println("Clap sound was detected!");
LED_state = !LED_state;
digitalWrite(LED_pin, LED_state ? HIGH : LOW);
}
last_event = millis();
}
}
Stepper motor controling sound sensor
Final Product
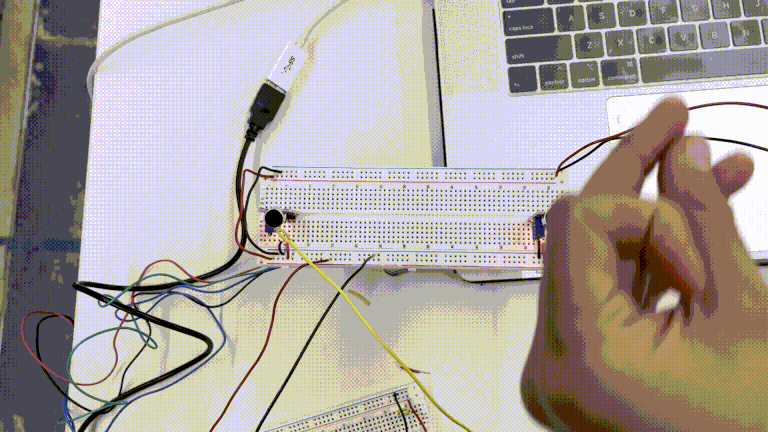
Wiring
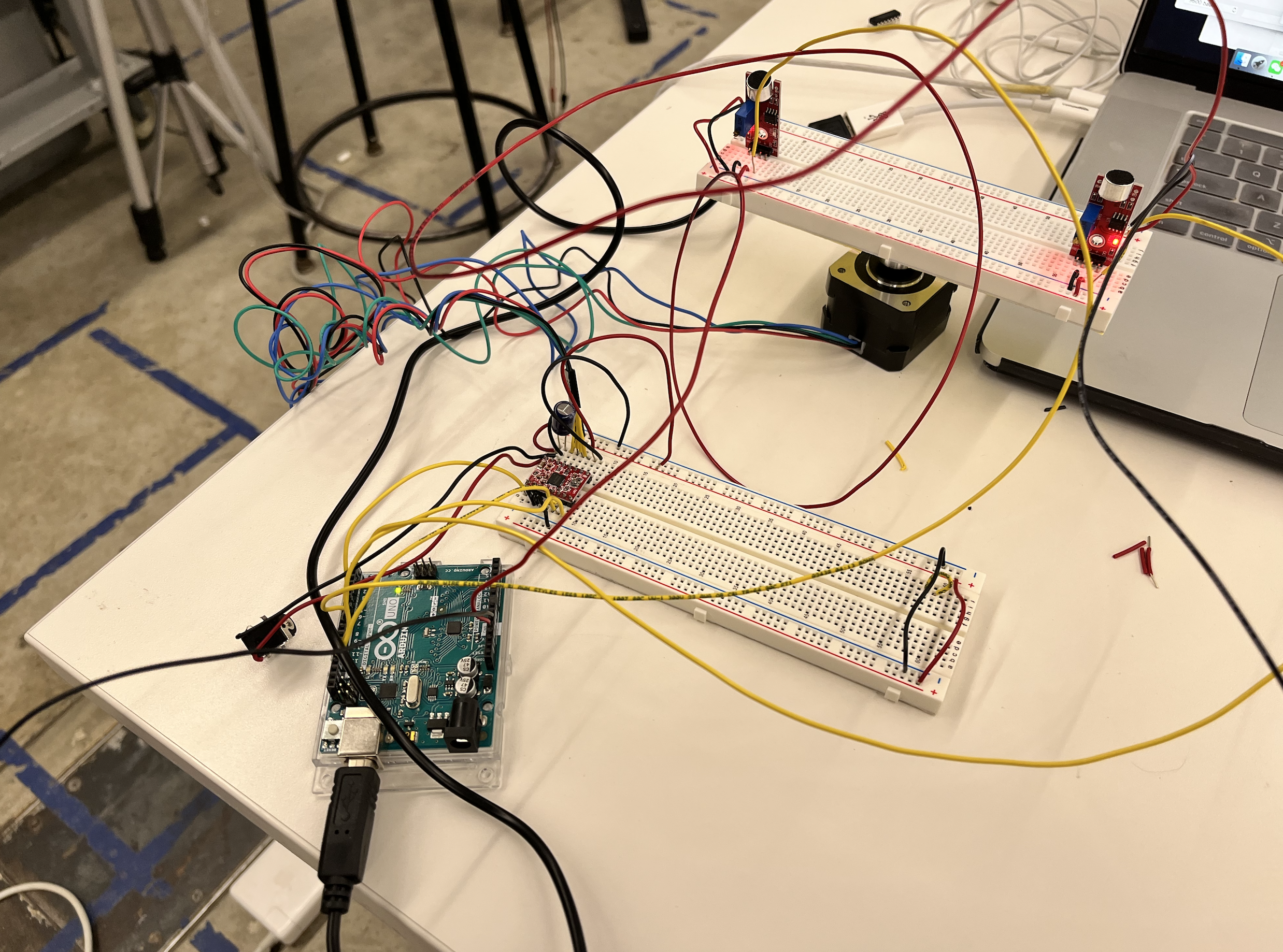
Arduino code for stepper motor sound sensor
const int stepsPerRevolution = 400; // change this to fit the number of steps per revolution for your motor
const int numberOfSteps = stepsPerRevolution/8; //45 degree turns
const int dirPin=12;
const int stepPin=13;
const int rightSensorPin=7;
const int leftSensorPin=8;
const int enablePin=5 ;
boolean rightVal = 0;
boolean leftVal = 0;
void setup()
{
pinMode(leftSensorPin, INPUT); //Make pin 8 an input pin.
pinMode(rightSensorPin, INPUT); //Make pin 7 an input pin.
pinMode (stepPin, OUTPUT); //Make pin 13 an output pin.
pinMode (dirPin, OUTPUT); //Make pin 12 an output pin.
pinMode (enablePin, OUTPUT); //Make pin 5 an output pin.
digitalWrite(enablePin, LOW); //Enable is active low
Serial.begin (9600); // initialize the serial port:
}
void loop ()
{
//poll inputs for signal
rightVal =digitalRead(rightSensorPin);
leftVal =digitalRead(leftSensorPin);
// when the sensor detects a signal above the threshold value set on sensor, turn finder to the direction of sound
if (leftVal==LOW && rightVal==HIGH)
{
Serial.println("Turning Right");
digitalWrite(dirPin,LOW); //turn counter-clockwise
//turn finder in the direction of the sound
for(int steps = 0; steps < numberOfSteps; steps++)
{
//create pulse to turn motor one step at a time
digitalWrite(stepPin,HIGH);
delayMicroseconds(10000);
digitalWrite(stepPin, LOW);
delayMicroseconds(10000);
}
delayMicroseconds(100000);
rightVal = 0;
leftVal = 0;
}
else if (leftVal==HIGH && rightVal==LOW)
{
Serial.println("Turning Left");
digitalWrite(dirPin,HIGH); //turn clockwise
//turn finder in the direction of the sound
for(int steps = 0; steps < numberOfSteps; steps++)
{
//create pulse to turn motor one step at a time
digitalWrite(stepPin,HIGH);
delayMicroseconds(10000);
digitalWrite(stepPin, LOW);
delayMicroseconds(10000);
}
delayMicroseconds(100000);
rightVal = 0;
leftVal = 0;
}
else
{
//Do nothing
rightVal = 0;
leftVal = 0;
}
}