LED lights
This week we learned about microcontroller. I find online tutorial on traffic light to be a good practice on this topic. I first did the arduino diagram and prepared everything I need.
Arduino Diagram
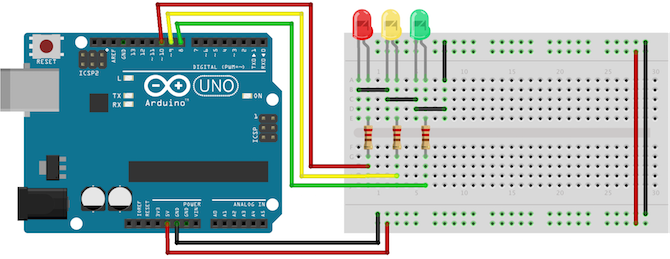
Equipment ready
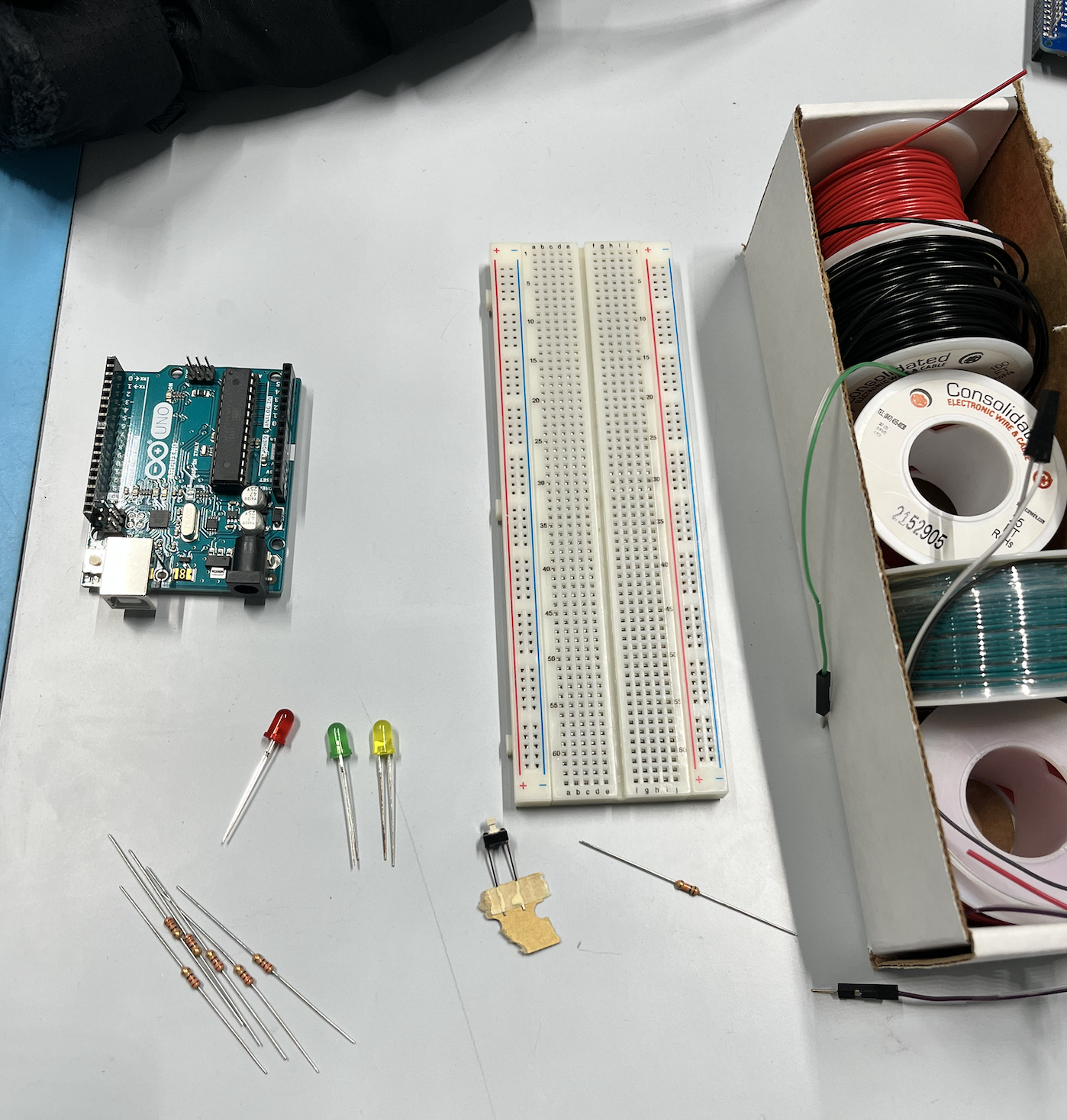
Equipment
- LED lights: Red, Yellow, Green
- 6 220 ohm Resistors
- Button
- Arduino Uno
- 10k ohm Resistors
- Wires
- Breadboard
Then I first connected with one pair of LED lights and it seems it works. I also tried to add a button to control it.
One Pair of LED lights
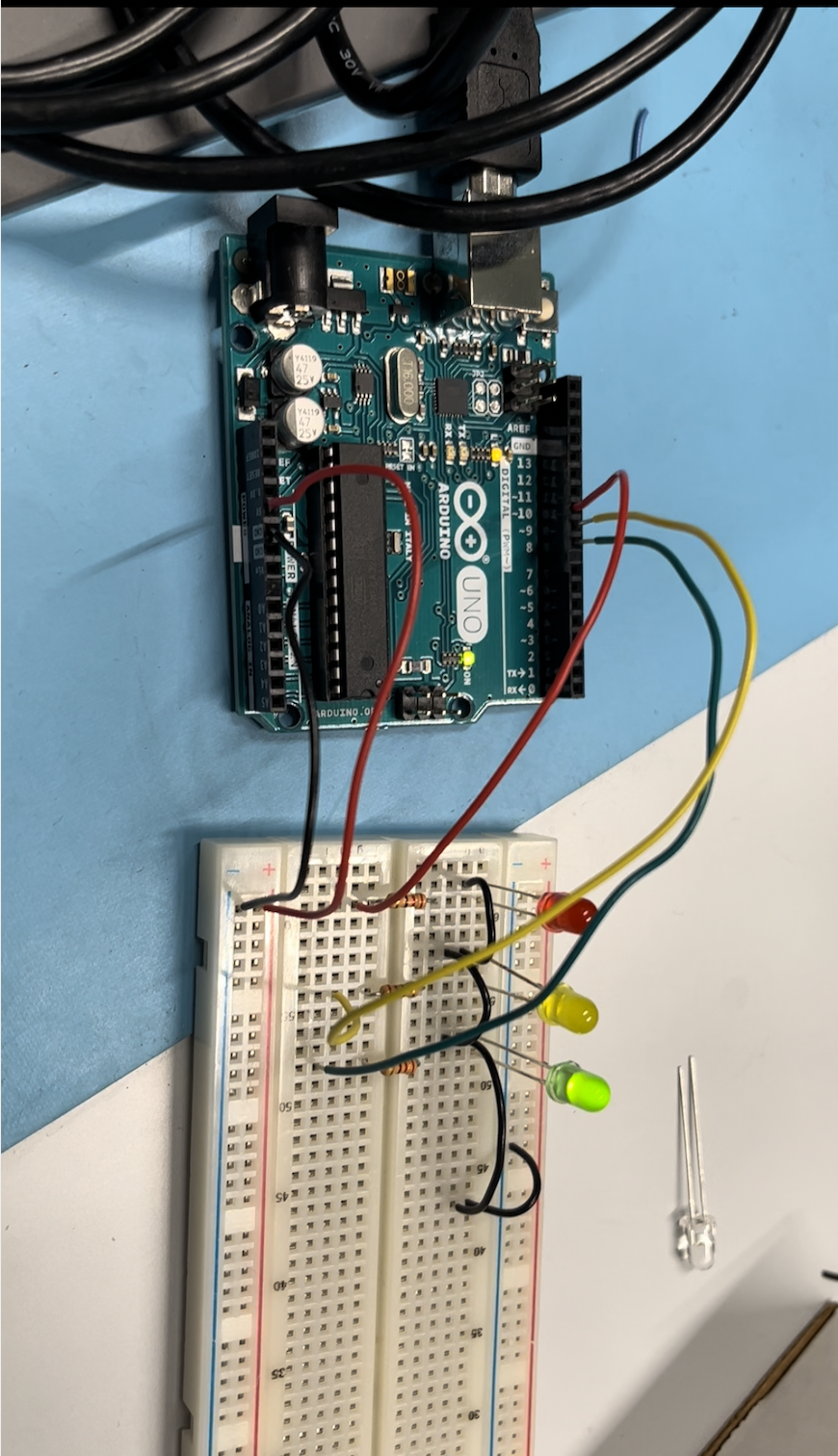
LED lights with button
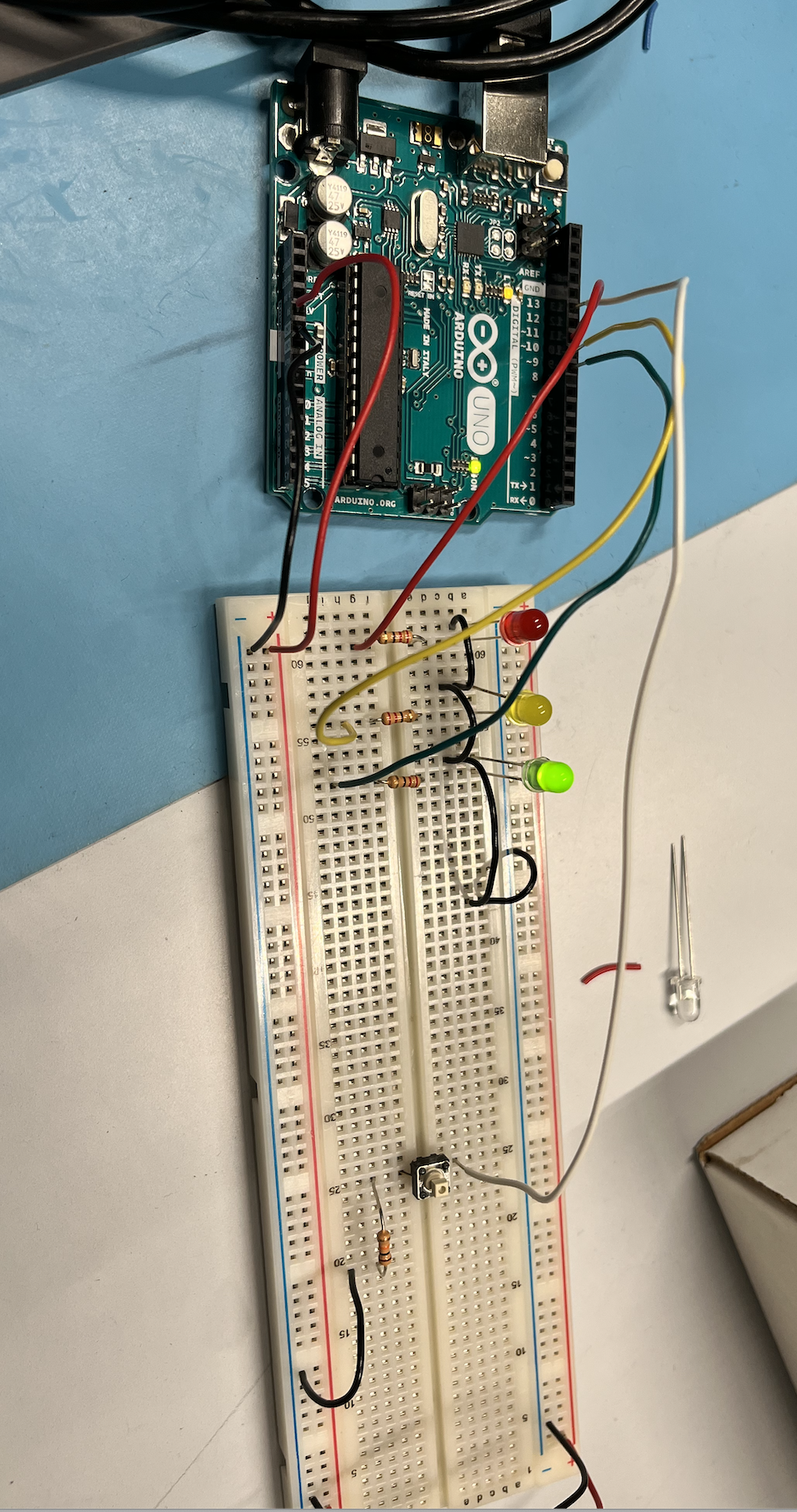
Final product
Then I put two pairs of LED lights together.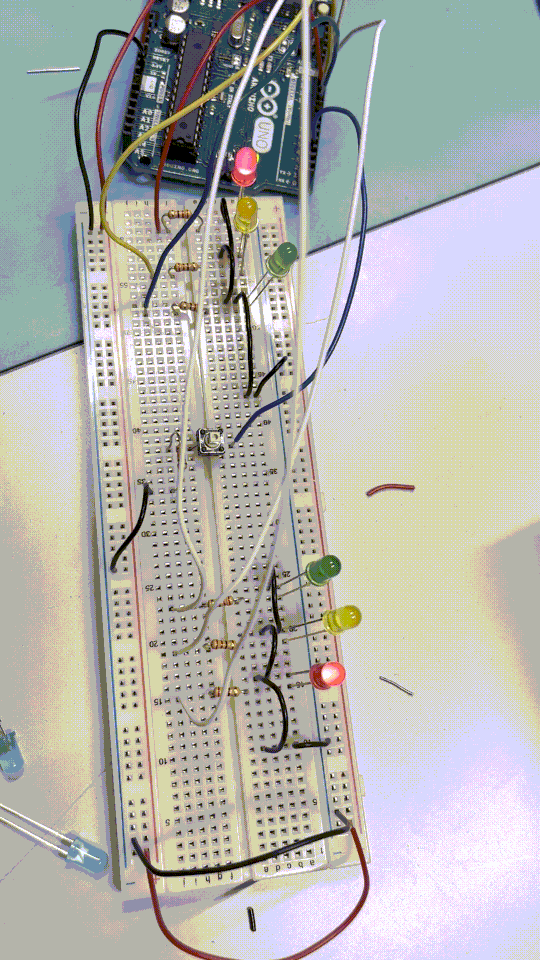
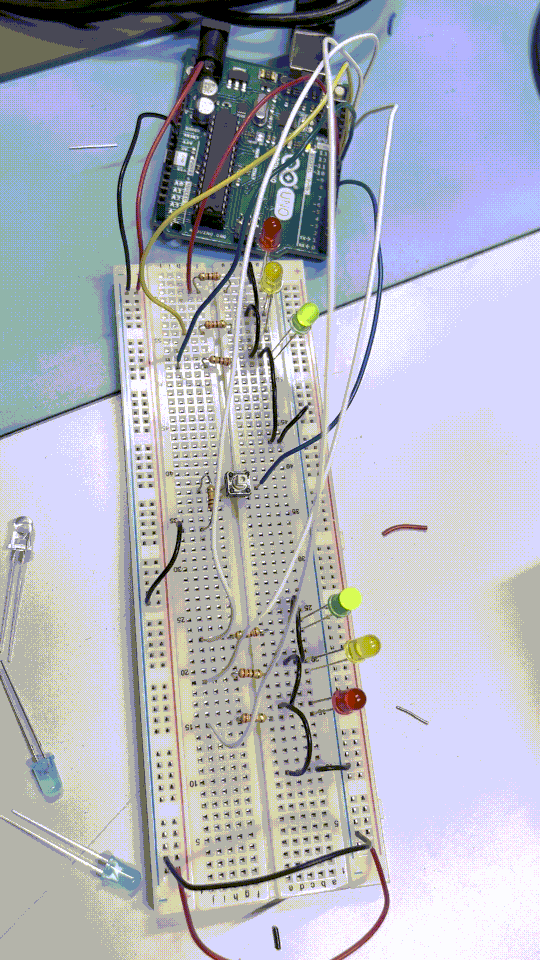
I also tried to play around with other LED lights and seems fun.
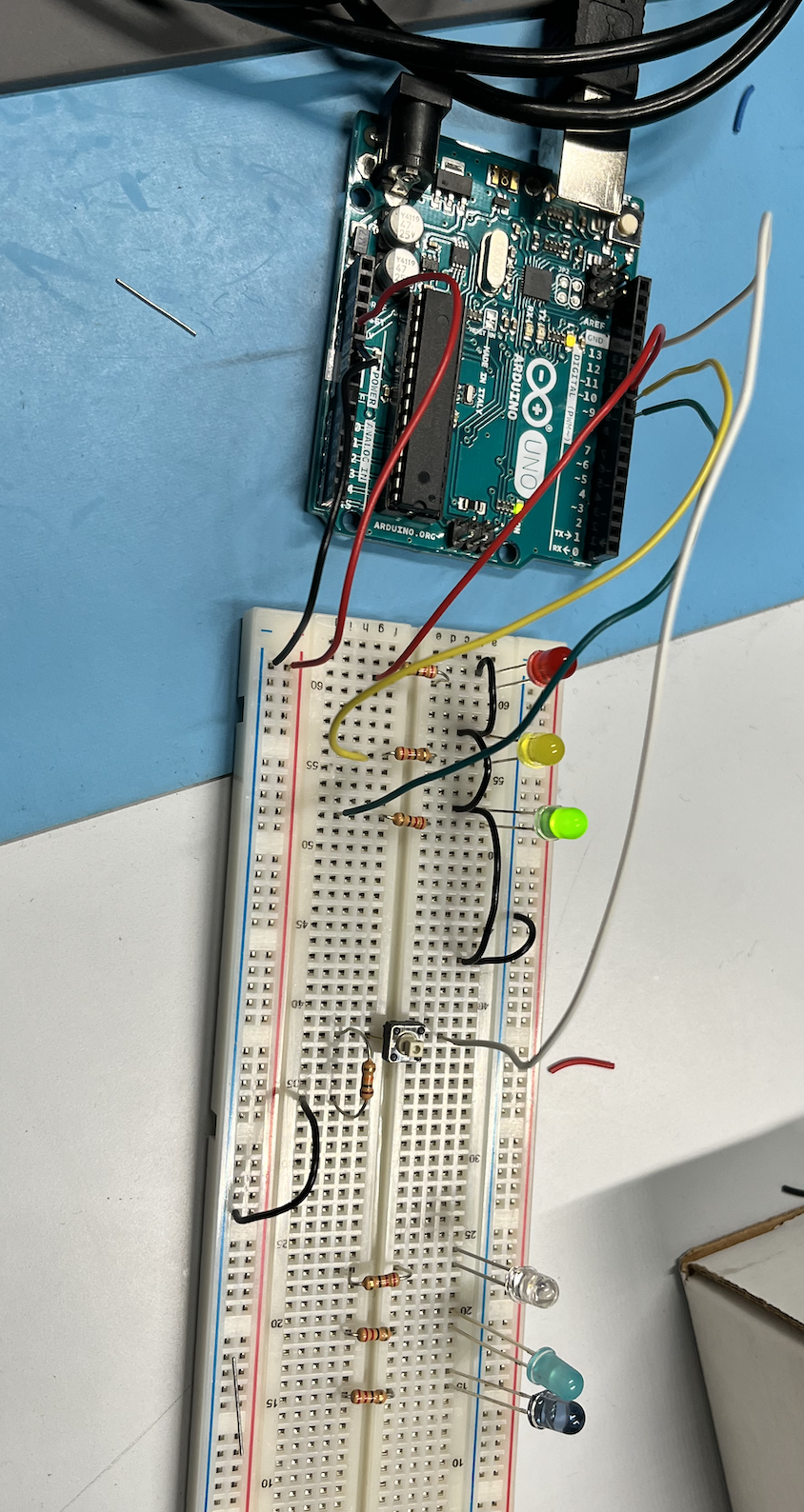
Arduino code
// light one
int red1 = 10;
int yellow1 = 9;
int green1 = 8;
// light two
int red2 = 13;
int yellow2 = 12;
int green2 = 11;
//button
int button = 3; // switch is on pin 3
void setup() {
// put your setup code here, to run once:
// light one
pinMode(red1, OUTPUT);
pinMode(yellow1, OUTPUT);
pinMode(green1, OUTPUT);
// light two
pinMode(red2, OUTPUT);
pinMode(yellow2, OUTPUT);
pinMode(green2, OUTPUT);
//button
pinMode(button, INPUT);
digitalWrite(green1, HIGH);
}
void loop() {
// put your main code here, to run repeatedly:
//original code
changeLights();
delay(1000);
//
//with button
// if (digitalRead(button) == HIGH){
// delay(15); // software debounce
// if (digitalRead(button) == HIGH) {
// // if the switch is HIGH, ie. pushed down - change the lights!
// changeLights();
// delay(15000); // wait for 15 seconds
// }
// }
//
}
//two lights
void changeLights(){
// turn both yellows on
digitalWrite(green1, LOW);
digitalWrite(yellow1, HIGH);
digitalWrite(yellow2, HIGH);
delay(2000);
// turn both yellows off, and opposite green and red
digitalWrite(yellow1, LOW);
digitalWrite(red1, HIGH);
digitalWrite(yellow2, LOW);
digitalWrite(red2, LOW);
digitalWrite(green2, HIGH);
delay(2000);
// both yellows on again
digitalWrite(yellow1, HIGH);
digitalWrite(yellow2, HIGH);
digitalWrite(green2, LOW);
delay(2000);
// turn both yellows off, and opposite green and red
digitalWrite(green1, HIGH);
digitalWrite(yellow1, LOW);
digitalWrite(red1, LOW);
digitalWrite(yellow2, LOW);
digitalWrite(red2, HIGH);
delay(2000);
}
//one light
//void changeLights(){
// // green off, yellow on for 3 seconds
// digitalWrite(green, LOW);
// digitalWrite(yellow, HIGH);
// delay(3000);
// // turn off yellow, then turn red on for 5 seconds
// digitalWrite(yellow, LOW);
// digitalWrite(red, HIGH);
// delay(5000);
// // red and yellow on for 2 seconds (red is already on though)
// digitalWrite(yellow, HIGH);
// delay(2000);
// // turn off red and yellow, then turn on green
// digitalWrite(yellow, LOW);
// digitalWrite(red, LOW);
// digitalWrite(green, HIGH);
// delay(3000);
//}